Configuration (part 3 – Registry)
In part 3 we’re again looking at how to use the API to write some code, this time for the registry solution.
Registry
The NA_RegistryService interface provides the registry functionality, and we’ll also be examining use of NA_Commanding to tell our targets to reload their configuration from the registry. For our example, we’ll use a serial channel and we’ll configure the following items,
- Rx Baud rate
- Sync pattern
- Frame length
First let’s talk about the registry keys that we need to know. We’ll be working on channel zero (0), so the base registry path for all these will be /netacq/server/device/sio/0/0.
- Rx Baud rate
- ibaudrat – floating point baud rate
- Sync pattern
- syncpat – space delimited, e.g., “FA F3 20”
- synclen – count, in bits, of the sync length
- Frame length
- iminlen – (bits) minimum frame length, sync with max (else means variable length)
- imaxlen – (bits) maximum frame length
- bufsize – (bytes) match to imaxlen, byte-integral
Commanding
Once we’ve set the key(s), we’ll need to command the channel to Reload so it re-reads the registry and picks up the new configuration.
UNC paths
The commanding object typically follows a path pattern similar to the registry area where the configuration is found, minus the prefix of /netacq/server.
// data flow 0
server.GetCommanding("/device/dfe/0");
// serial channel 5
server.GetCommanding("/device/sio/0/5");
// recorder 3
server.GetCommanding("/dispatch/p_recorder/3");
Commanding nuances
Committing and commanding an entity differs slightly depending on what the entity does. Think of entities as they fall into one of two categories: “playable” types and those that just operate.
Playable entities
Subsystems that are controllable with notions of stop/start need to be stopped and started again. These entities include (non-exhaustive list),
- Recorder
- Player
- BERT
- SIOGEN
However, Data Flow is not included in this type.
Other entities
Other entities such as serial, data flow, etc., can simply be reloaded via “Reload” command to force a re-read of the registry and reconfiguration.
// serial channel 5
NA_Commanding ch5 = server.GetCommanding("/device/sio/0/5");
ch5.CommandEntity("Reload");
Putting it all together
Let’s look at some examples.
Serial channel configuration
Here’s our before,
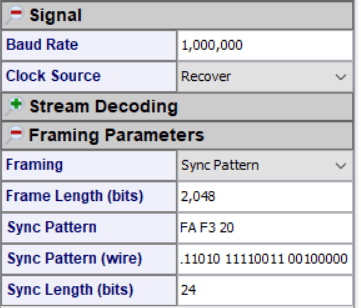
string ch0 = "/netacq/server/device/sio/0/0/";
NA_RegistryService registry = server.GetRegistry();
registry.SetString(ch0 + "ibaudrat", "2000000");
registry.SetString(ch0 + "syncpat", "d2b3c6a1");
registry.SetString(ch0 + "synclen ", "32");
registry.SetString(ch0 + "iminlen", "4096");
registry.SetString(ch0 + "imaxlen", "4096");
registry.SetString(ch0 + "bufsize", "512");
// or using batch - 1 write operation
KeyValuePair[] keys = {
new KeyValuePair(ch0 + "ibaudrat", "2000000"),
new KeyValuePair(ch0 + "syncpat", "d2 b3 c6 a1"),
new KeyValuePair(ch0 + "synclen ", "32"),
new KeyValuePair(ch0 + "iminlen", "4096"),
new KeyValuePair(ch0 + "imaxlen", "4096"),
new KeyValuePair(ch0 + "bufsize", "512"),
};
// (keys, atomic_operation, create_if_missing, abort_if_exists)
registry.WriteKeys(keys, true, true, false);
// then reload the channel to complete
NA_Commanding ch0Cmd = server.GetCommanding("/device/sio/0/0");
ch0Cmd.CommandEntity("Reload");
And our after,
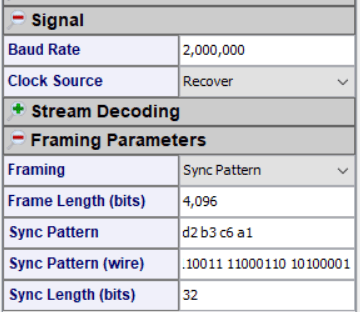
Recorder configuration
Let’s try a recorder example. We’ll start with a new, empty recorder instance.
string recorder0 = "/netacq/server/dispatch/p_recorder/0/";
NA_RegistryService registry = server.GetRegistry();
// special format: [channel#, UNC, type)
registry.SetString(recorder0 + "streams", "1 sio/0/in0 Throughput");
registry.SetString(recorder0 + "recordingname", "example");
// raw binary recording
registry.SetString(recorder0 + "recordingformat", "1");
// or using batch - 1 write operation
KeyValuePair[] keys = {
new KeyValuePair(recorder0 + "streams", "1 sio/0/in0 Throughput"),
new KeyValuePair(recorder0 + "recordingname", "example"),
new KeyValuePair(recorder0 + "recordingformat", "1")
};
// (keys, atomic_operation, create_if_missing, abort_if_exists)
registry.WriteKeys(keys, true, true, false);
NA_Commanding recorder0Cmd = server.GetCommanding("dispatch/p_recorder/0");
// NOTE: some entities enforce states, we'll assume it's running for simplicity
recorder0Cmd.CommandEntity("Stop");
recorder0Cmd.CommandEntity("Start");
And our after,
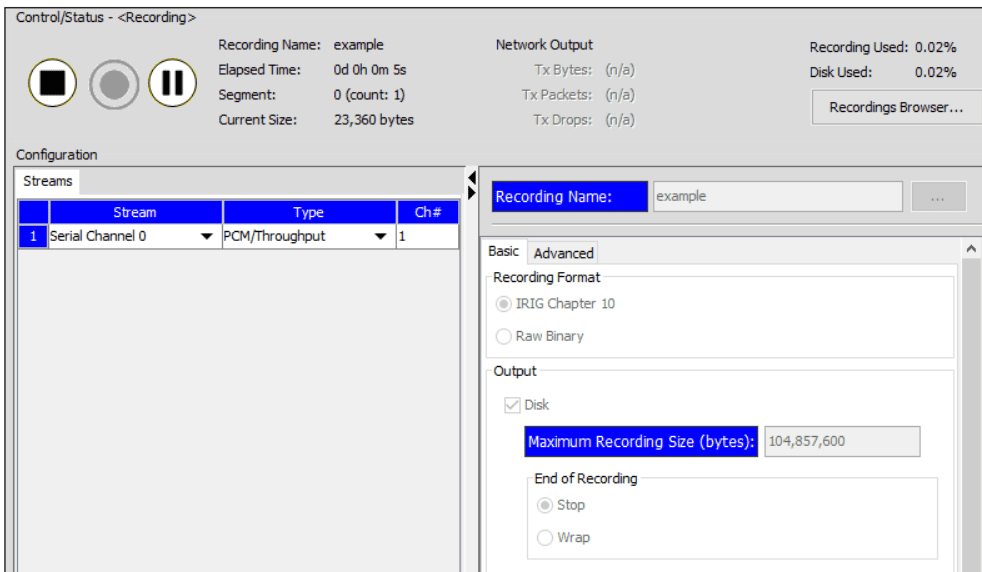